Practical Go
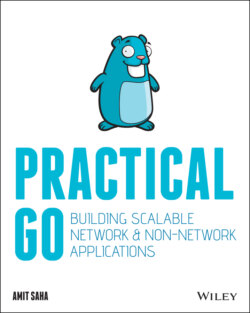
Реклама. ООО «ЛитРес», ИНН: 7719571260.
Оглавление
Amit Saha. Practical Go
Table of Contents
List of Tables
List of Illustrations
Guide
Pages
Practical Go. Building Scalable Network and Non-Network Applications
Introduction
What Does This Book Cover?
Reader Support for This Book
Getting Started
Installing Go
Choosing an Editor
Installing Protocol Buffer Toolchain
Linux and macOS
Windows
Installing Docker Desktop
Guide to the Book
Go Modules
Command Line and Terminals
Terms
Robustness and Resiliency
Production Readiness
Reference Documentation
Go Refresher
Struct Type
Interface Type
Goroutines and Channels
Testing
Summary
CHAPTER 1 Writing Command-Line Applications
Your First Application
Listing 1.1: A greeter application
Writing Unit Tests
Listing 1.2: Test for the parseArgs() function
Listing 1.3: Test for the validateArgs() function
Listing 1.4: Test for the runCmd() function
Using the Flag Package
Listing 1.5: Greeter using flag
Testing the Parsing Logic
Listing 1.6: Test for the parseArgs() function
Improving the User Interface
Removing Duplicate Error Messages
Customizing Usage Message
Accept Name via a Positional Argument
Listing 1.7: Greeter program with user interface updates
Updating the Unit Tests
Listing 1.8: Test for parseArgs() function
Listing 1.9: Test for runCmd() function
Summary
CHAPTER 2 Advanced Command-Line Applications
Implementing Sub-commands
Listing 2.1: Implementing sub-commands in a command-line application
An Architecture for Sub-command-Driven Applications
Listing 2.2: Implementation of the main package
Listing 2.3: Implementation of the HandleHttp() function
Listing 2.4: Implementation of the HandleGrpc() function
Listing 2.5: Custom error values
Testing the Main Package
Listing 2.6: Unit test for the main package
Testing the Cmd Package
Making Your Applications Robust
User Input with Deadlines
Listing 2.7: Implementing time-out for user input
Handling User Signals
Listing 2.8: Handling user signals
Summary
CHAPTER 3 Writing HTTP Clients
Downloading Data
Listing 3.1: A basic data downloader
Testing the Data Downloader
Listing 3.2: Test for fetchRemoteResource() function
Deserializing Received Data
Listing 3.3: Querying data from the package server
Listing 3.4: Test for pkgquery
Sending Data
Listing 3.5: Register a new package
Listing 3.6: Test for registering a new package
Working with Binary Data
Listing 3.7: Creating a multipart message
Listing 3.8: Package registration using multipart message
Listing 3.9: Test for package registration with multipart message
Summary
CHAPTER 4 Advanced HTTP Clients
Using a Custom HTTP Client
Downloading from an Overloaded Server
Listing 4.1: Test for fetchRemoteResource() function with a bad server
Listing 4.2: The data downloader application with a custom HTTP client with time-out
Testing the Time-Out Behavior
Listing 4.3: Test for fetchRemoteResource() function with a bad server
Listing 4.4: Test for fetchRemoteResource() function with the updated bad server
Configuring the Redirect Behavior
Listing 4.5: A data downloader that exits if there is a redirect attempt
Customizing Your Requests
Implementing Client Middleware
Understanding the RoundTripper Interface
A Logging Middleware
Listing 4.6: A data downloader with a custom logging middleware
Add a Header to All Requests
Listing 4.7: An HTTP client with a middleware to add custom headers
Listing 4.8: Test for middleware to add headers
Connection Pooling
Listing 4.9: A program to illustrate connection pooling
Configuring the Connection Pool
Summary
CHAPTER 5 Building HTTP Servers
Your First HTTP Server
Listing 5.1: A basic HTTP server
Setting Up Request Handlers
Handler Functions
Listing 5.2: An HTTP server using a dedicated ServeMux object
Testing Your Server
Listing 5.3: A test for the HTTP server
The Request Struct
Method
URL
Proto, ProtoMajor, and ProtoMinor
Header
Host
Body
Form, PostForm
MultipartForm
Attaching Metadata to a Request
Listing 5.4: Attaching metadata to a request
Processing Streaming Requests
Listing 5.5: Decoding JSON data using Decode()
Streaming Data as Responses
Listing 5.6: Streaming response
Summary
CHAPTER 6 Advanced HTTP Server Applications
The Handler Type
Sharing Data across Handler Functions
Listing 6.1: An HTTP server using a custom handler type
Writing Server Middleware
Custom HTTP Handler Technique
The HandlerFunc Technique
Chaining Middleware
Listing 6.2: Chaining middleware using http.HandleFunc
Writing Tests for Complex Server Applications
Code Organization
Listing 6.3: Managing application configuration
Listing 6.4: Request handlers
Listing 6.5: Setting up the request handlers
Listing 6.6: Logging and panic-handling middleware
Listing 6.7: Middleware registration
Listing 6.8: Main server
Testing the Handler Functions
Listing 6.9: Test for the API handler function
Testing the Middleware
Listing 6.10: Test for panic-handling middleware
Testing the Server Startup
Listing 6.11: Test for server setup
Summary
CHAPTER 7 Production-Ready HTTP Servers
Aborting Request Handling
Listing 7.1: Enforcing time-out on a handler function
Strategies to Abort Request Processing
Listing 7.2: Enforcing time-out on a handler function
Handling Client Disconnects
Listing 7.3: Handling client disconnects
Server-Wide Time-Outs
Implement a Time-Out for All Handler Functions
Implementing Server Time-Out
Listing 7.4: Configuring server time-outs
Implementing Graceful Shutdown
Listing 7.5: Implementing graceful shutdown in a server
Securing Communication with TLS
Configuring TLS and HTTP/2
Listing 7.6: Securing an HTTP server using TLS
Testing TLS Servers
Listing 7.7: Securing an HTTP server using TLS with a configurable logger
Listing 7.8: Verify middleware behavior in a TLS-enabled HTTP server
Summary
CHAPTER 8 Building RPC Applications with gRPC
gRPC and Protocol Buffers
Writing Your First Service
Listing 8.1: Protobuf specification for Users service
Writing the Server
Listing 8.2: gRPC server for the Users service
Listing 8.3: go.mod file for the Users gRPC server
Writing a Client
Listing 8.4: Client for the Users service
Listing 8.5: go.mod file for the Users service client
Testing the Server
Listing 8.6: Test for the Users service
Testing the Client
Listing 8.7: Test for the Users service client
A Detour into Protobuf Messages
Marshalling and Unmarshalling
Listing 8.8: Client for the Users service
Listing 8.9: go.mod file for the Users service client supporting a JSON-formatted query
Listing 8.10: Client for the Users service working with JSON and protobuf
Forward and Backward Compatibility
Multiple Services
Listing 8.11: Protobuf specification for the Repo service
Listing 8.12: gRPC Server with both the Users and Repo services
Listing 8.13: go.mod file for gRPC service with the User and Repo services
Error Handling
Summary
CHAPTER 9 Advanced gRPC Applications
Streaming Communication
Server-Side Streaming
Listing 9.1: Protobuf specification for the Repo service
Listing 9.2: gRPC server for Users and Repo service
Listing 9.3: go.mod file for the server
Listing 9.4: Test function for the Repo service
Client-Side Streaming
Bidirectional Streaming
Listing 9.5: Protobuf specification for the Users service
Listing 9.6: Server for the Users service
Listing 9.7: go.mod file for the server
Listing 9.8: Client for the Users service
Listing 9.9: go.mod file for the client
Receiving and Sending Arbitrary Bytes
Listing 9.10: Protobuf specification for the Repo service
Listing 9.11: Server for the Repo service
Listing 9.12: go.mod file for the Repo service server implementation
Implementing Middleware Using Interceptors
Listing 9.13: go.mod file for the Users service
Listing 9.14: go.mod file for the Users server
Listing 9.15: go.mod file for the Users client
Client-Side Interceptors
Listing 9.16: Updated protobuf specification for the Users service
Listing 9.17: Client application for the Users service with interceptors
Server-Side Interceptors
Listing 9.18: Server application for the Users service with interceptors
Wrapping Streams
Chaining Interceptors
Summary
CHAPTER 10 Production-Ready gRPC Applications
Securing Communication with TLS
Robustness in Servers
Implementing Health Checks
Handling Runtime Errors
Aborting Request Processing
Robustness in Clients
Improving Connection Setup
Handling Transient Failures
Setting Time-Outs for Method Calls
Connection Management
Summary
CHAPTER 11 Working with Data Stores
Working with Object Stores
Integration with Package Server
Testing Package Uploads
Accessing Underlying Driver Types
Working with Relational Databases
Integration with Package Server
Testing Data Storage
Data Type Conversions
Using Database Transactions
Summary
APPENDIX A Making Your Applications Observable
Logs, Metrics, and Traces
Emitting Telemetry Data
Command-Line Applications
HTTP Applications
gRPC Applications
Summary
APPENDIX B Deploying Applications
Managing Configuration
Distributing Your Application
Deploying Server Applications
Summary
Index
About the Author
About the Technical Editor
Acknowledgments
WILEY END USER LICENSE AGREEMENT
Отрывок из книги
NOTE A glossary of relevant terms is available for free download from the book's web page: https://www.wiley.com/go/practicalgo.
My goal in this book is to showcase the various features of the Go language and the standard libraries (along with a few community-maintained packages) by developing various categories of applications. Once you have refreshed or learned the language fundamentals, this book will help you take the next step. I have adopted a writing style where the focus is on using various features of the language and its libraries to solve the particular problem at hand—one that you care about.
.....
The function takes two parameters: a variable, w, whose value satisfies the io.Writer interface, and an array of strings representing the arguments to parse. It returns a config object and an error value. To parse the arguments, a new FlagSet object is created as follows:
fs := flag.NewFlagSet("greeter", flag.ContinueOnError)
.....