Programming Kotlin Applications
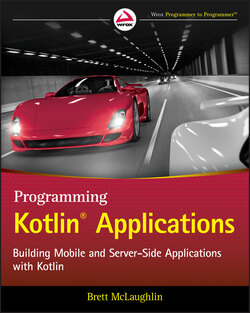
Реклама. ООО «ЛитРес», ИНН: 7719571260.
Оглавление
Бретт Мак-Лахлин. Programming Kotlin Applications
Table of Contents
List of Tables
List of Illustrations
Guide
Pages
Programming Kotlin® Applications. BUILDING MOBILE AND SERVER-SIDE APPLICATIONS WITH KOTLIN
ABOUT THE AUTHOR
ABOUT THE TECHNICAL EDITOR
ACKNOWLEDGMENTS
INTRODUCTION
WHAT DOES THIS BOOK COVER?
WILL THIS BOOK TEACH ME TO PROGRAM MOBILE APPLICATIONS IN KOTLIN?
Reader Support for This BookCompanion Download Files
How to Contact the Publisher
How to Contact the Author
1 Objects All the Way Down. WHAT'S IN THIS CHAPTER?
KOTLIN: A NEW PROGRAMMING LANGUAGE
LISTING 1.1: A simple Kotlin program using classes and lists
WHAT IS KOTLIN?
What Does Kotlin Add to Java?
KOTLIN IS OBJECT-ORIENTED
LISTING 1.2: A very useless object in Kotlin (and a main function to use it)
INTERLUDE: SET UP YOUR KOTLIN ENVIRONMENT
Install Kotlin (and an IDE)
Install IntelliJ
Create Your Kotlin Program
Compile and Run Your Kotlin Program
Fix Any Errors as They Appear
Install Kotlin (and Use the Command Line)
Command-Line Kotlin on Windows
Command-Line Kotlin on Mac OS X
Command-Line Kotlin on UNIX-Based Systems
Verify Your Command-Line Installation
CREATING USEFUL OBJECTS
Pass In Values to an Object Using Its Constructor
LISTING 1.3: A less useless object in Kotlin and its constructor
LISTING 1.4: Cutting out the constructor keyword
Print an Object with toString()
Terminology Update: Functions and Methods
Print an Object (and Do It with Shorthand)
Override the toString() Method
All Data Is Not a Property Value
LISTING 1.5: Converting data to actual properties
INITIALIZE AN OBJECT AND CHANGE A VARIABLE
LISTING 1.6: Creating a new property for a Person
Initialize a Class with a Block
Kotlin Auto-Generates Getters and Setters
Terminology Update: Getters, Setters, Mutators, Accessors
Constants Can't Change (Sort of)
LISTING 1.7 Using mutable variables
2 It's Hard to Break Kotlin. WHAT'S IN THIS CHAPTER?
UPGRADE YOUR KOTLIN CLASS GAME
Name a File According to Its Class
LISTING 2.1: The Person code from Chapter 1
LISTING 2.2: Main function for testing the Person class
Organize Your Classes with Packages
Put Person in a Package
Classes: The Ultimate Type in Kotlin
KOTLIN HAS A LARGE NUMBER OF TYPES
Numbers in Kotlin
Letters and Things
Truth or Fiction
Types Aren't Interchangeable (Part 1)
LISTING 2.3: Person with extra properties
You Must Initialize Your Properties
Types Aren't Interchangeable (Part 2)
LISTING 2.4: Filling out your Person instances
You Can Explicitly Tell Kotlin What Type to Use
Try to Anticipate How Types Will Be Used
It's Easy to Break Kotlin (Sort of)
OVERRIDING PROPERTY ACCESSORS AND MUTATORS
Custom-Set Properties Can't Be in a Primary Constructor
Move Properties Out of Your Primary Constructors
Initialize Properties Immediately
LISTING 2.5: Assigning constructor information to properties
Try to Avoid Overusing Names
LISTING 2.6: Clarifying property names and constructor inputs
Override Mutators for Certain Properties
LISTING 2.7: Defining custom mutators for lastName and firstName
CLASSES CAN HAVE CUSTOM BEHAVIOR
Define a Custom Method on Your Class
LISTING 2.8: Creating a new property method to update the fullName variable
Every Property Must Be Initialized
Assign an Uninitialized Property a Dummy Value
Tell Kotlin You'll Initialize a Property Later
Assign Your Property the Return Value from a Function
Listing 2.9 Getting the fullName property correct (partially)
Sometimes You Don't Need a Property!
LISTING 2.10: A much simpler version of Person
TYPE SAFETY CHANGES EVERYTHING
WRITING CODE IS RARELY LINEAR
3 Kotlin Is Extremely Classy. WHAT'S IN THIS CHAPTER?
OBJECTS, CLASSES, AND KOTLIN
ALL CLASSES NEED AN EQUALS(X) METHOD
Equals(x) Is Used to Compare Two Objects
LISTING 3.1: Using equals(x) to compare two instances
Override equals(x) to Make It Meaningful
Every Object Is a Particular Type
LISTING 3.2: Person with a working equals(x) override
LISTING 3.3: Testing out equals(x) in a few scenarios
A Brief Introduction to Null
EVERY OBJECT INSTANCE NEEDS A UNIQUE HASHCODE()
All Classes Inherit from Any
LISTING 3.4: Any, the base class for all Kotlin classes
Always Override hashCode() and equals(x)
LISTING 3.5: Updating the test class to allow for comparing equals results with hash code results
Default Hash Codes Are Based on Memory Location
Use Hash Codes to Come Up with Hash Codes
SEARCHING (AND OTHER THINGS) DEPEND ON USEFUL AND FAST EQUALS(X) AND HASHCODE()
Multiple Properties to Differentiate Them in hashCode()
Use == over equals(x) for Speed
A Quick Speed Check on hashCode()
BASIC CLASS METHODS ARE REALLY IMPORTANT
4 Inheritance Matters. WHAT'S IN THIS CHAPTER?
GOOD CLASSES ARE NOT ALWAYS COMPLEX CLASSES
Keep It Simple, Stupid
LISTING 4.1: CardViewActivity
Keep It Flexible, Stupid
LISTING 4.2: Basic class to “draw” a square
LISTING 4.3: Extending Square to become a rectangle
LISTING 4.4: Reworking Square to use a more flexible property name
LISTING 4.5: Now extending Square is much cleaner
CLASSES CAN DEFINE DEFAULT VALUES FOR PROPERTIES
LISTING 4.6: The latest version of Person works, but isn't flexible
Constructors Can Accept Default Values
Kotlin Expects Arguments in Order
Specify Arguments by Name
Change the Order of Arguments (If You Need)
SECONDARY CONSTRUCTORS PROVIDE ADDITIONAL CONSTRUCTION OPTIONS
Secondary Constructors Come Second
Secondary Constructors Can Assign Property Values
LISTING 4.7: Current version of Person (with some problems!)
You Can Assign null to a Property … Sometimes
LISTING 4.8: Person with a working secondary constructor
LISTING 4.9: Testing out the secondary constructor of Person
null Properties Can Cause Problems
HANDLE DEPENDENT VALUES WITH CUSTOM MUTATORS
Set Dependent Values in a Custom Mutator
All Property Assignments Use the Property's Mutator
Nullable Values Can Be Set to null!
LISTING 4.10: Person with updated secondary constructor and a custom mutator
Limit Access to Dependent Values
When Possible, Calculate Dependent Values
LISTING 4.11: Update Person to have a new method, hasPartner(), and remove the property of the same name
You Can Avoid Parentheses with a Read-Only Property
LISTING 4.12: Moving from a function back to a property, but this time, a read-only one
LISTING 4.13: The PersonTest class has a helper function and works with the updated Person class
NEED SPECIFICS? CONSIDER A SUBCLASS
Any Is the Base Class for Everything in Kotlin
LISTING 4.14: Any, the base class for all Kotlin classes
{ … } Is Shorthand for Collapsed Code
A Class Must Be Open for Subclassing
LISTING 4.15: Parent, an eventual subclass of Person
Terminology: Subclass, Inherit, Base Class, and More
A Subclass Must Follow Its Superclass's Rules
A Subclass Gets Behavior from All of Its Superclasses
YOUR SUBCLASS SHOULD BE DIFFERENT THAN YOUR SUPERCLASS
Subclass Constructors Often Add Arguments
Don't Make Mutable What Isn't Mutable
LISTING 4.16: Updated test class
Sometimes Objects Don't Exactly Map to the Real World
Generally, Objects Should Map to the Real World
5 Lists and Sets and Maps, Oh My! WHAT'S IN THIS CHAPTER?
LISTS ARE JUST A COLLECTION OF THINGS
Kotlin Lists: One Type of Collection
LISTING 5.1: Beginning to play with lists
Collection Is a Factory for Collection Objects
Collection Is Automatically Available to Your Code
Mutating a Mutable List
Getting Properties from a Mutable List
LISTING 5.2: Working with mutable lists through basic methods
LISTS (AND COLLECTIONS) CAN BE TYPED
Give Your Lists Types
LISTING 5.3: Creating a list with a type
Iterate over Your Lists
LISTING 5.4: Very simple new class to represent a Band
LISTING 5.5: Reworking CollectionTest to use the new Band class
Kotlin Tries to Figure Out What You Mean
LISTS ARE ORDERED AND CAN REPEAT
Order Gives You Ordered Access
Lists Can Contain Duplicate Items
SETS: UNORDERED BUT UNIQUE
In Sets, Ordering Is Not Guaranteed
When Does Order Matter?
Sort Lists (and Sets) on the Fly
Sets: No Duplicates, No Matter What
Sets “Swallow Up” Duplicates
Sets Use equals(x) to Determine Existing Membership
LISTING 5.6: Updated equals(x) and hashCode() to improve Band
Using a Set? Check equals(x)
Iterators Aren't (Always) Mutable
MAPS: WHEN A SINGLE VALUE ISN'T ENOUGH
Maps Are Created by Factories
Use Keys to Find Values
How Do You Want Your Value?
FILTER A COLLECTION BY … ANYTHING
Filter Based on a Certain Criterion
Filter Has a Number of Useful Variations
COLLECTIONS: FOR PRIMITIVE AND CUSTOM TYPES
Add a Collection to Person
LISTING 5.7: The Person class from Chapter 4 (and before)
LISTING 5.8: The Parent class currently supports only a single child
Allow Collections to Be Added to Collection Properties
LISTING 5.9: Flipping constructors in Parent to function as they should
Sets and MutableSets Aren't the Same
Collection Properties Are Just Collections
6 The Future (in Kotlin) Is Generic. WHAT'S IN THIS CHAPTER?
GENERICS ALLOW DEFERRING OF A TYPE
Collections Are Generic
LISTING 6.1: Key bits of ArrayList source code
Parameterized Types Are Available Throughout a Class
Generic: What Exactly Does It Refer To?
GENERICS TRY TO INFER A TYPE WHEN POSSIBLE
Kotlin Looks for Matching Types
Kotlin Looks for the Narrowest Type
Sometimes Type Inference Is Wrong
Don't Assume You Know Object Intent
Kotlin Doesn't Tell You the Generic Type
Just Tell Kotlin What You Want!
COVARIANCE: A STUDY IN TYPES AND ASSIGNMENT
What about Generic Types?
LISTING 6.2: New Child class for use in type testing and experimentation
LISTING 6.3: New test class to look at variance in Kotlin
Some Languages Take Extra Work to Be Covariant
Kotlin Actually Takes Extra Work to Be Covariant, Too
Sometimes You Have to Make Explicit What Is Obvious
Covariant Types Limit the Input Type as Well as the Output Type
Covariance Is Really about Making Inheritance Work the Way You Expect
CONTRAVARIANCE: BUILDING CONSUMERS FROM GENERIC TYPES
Contravariance: Limiting What Comes Out Rather Than What Comes In
LISTING 6.4: The Comparator interface in Kotlin is contravariant
LISTING 6.5: The Comparator interface in Kotlin is contravariant
Contravariance Works from a Base Class Down to a Subclass
Contravariant Classes Can't Return a Generic Type
Does Any of This Really Matter?
UNSAFEVARIANCE: LEARNING THE RULES, THEN BREAKING THEM
TYPEPROJECTION LETS YOU DEAL WITH BASE CLASSES
Variance Can Affect Functions, Not Just Classes
Type Projection Tells Kotlin to Allow Subclasses as Input for a Base Class
Producers Can't Consume and Consumers Can't Produce
Variance Can't Solve Every Problem
7 Flying through Control Structures. WHAT'S IN THIS CHAPTER?
CONTROL STRUCTURES ARE THE BREAD AND BUTTER OF PROGRAMMING
IF AND ELSE: THE GREAT DECISION POINT
LISTING 7.1: A very basic usage of if and else
!! Ensures Non-Nullable Values
Control Structures Affect the Flow of Your Code
if and else Follow a Basic Structure
Expressions and if Statements
Use the Results of an if Statement Directly
Kotlin Has No Ternary Operator
A Block Evaluates to the Last Statement in That Block
if Statements That Are Assigned Must Have else Blocks
WHEN IS KOTLIN'S VERSION OF SWITCH
Each Comparison or Condition Is a Code Block
Handle Everything Else with an else Block
Each Branch Can Support a Range
Each Branch Usually Has a Partial Expression
Branch Conditions Are Checked Sequentially
Branch Conditions Are Just Expressions
When Can Be Evaluated as a Statement, Too
FOR IS FOR LOOPING
For in Kotlin Requires an Iterator
You Do Less, Kotlin Does More
For Has Requirements for Iteration
You Can Grab Indices Instead of Objects with for
LISTING 7.2: All the code snippets so far, in one sample program
USE WHILE TO EXECUTE UNTIL A CONDITION IS FALSE
While Is All about a Boolean Condition
A Wrinkle in while: Multiple Operators, One Variable
Combine Control Structures for More Interesting Solutions
DO … WHILE ALWAYS RUNS ONCE
Every do … while Loop Can Be Written as a while Loop
If Something Must Happen, Use do … while
LISTING 7.3: More code, more loops, more control structures
do … while Can Be a Performance Consideration
GET OUT OF A LOOP IMMEDIATELY WITH BREAK
Break Skips What's Left in a Loop
You Can Use a Label with break
GO TO THE NEXT ITERATION IMMEDIATELY WITH CONTINUE
Continue Works with Labels as Well
If versus continue: Mostly Style over Substance
RETURN RETURNS
8 Data Classes. WHAT'S IN THIS CHAPTER?
CLASSES IN THE REAL WORLD ARE VARIED BUT WELL EXPLORED
Many Classes Share Common Characteristics
LISTING 8.1: Revisiting the Person class
Common Characteristics Result in Common Usage
A DATA CLASS TAKES THE WORK OUT OF A CLASS FOCUSED ON DATA
LISTING 8.2: A new User class as a data class
Data Classes Handle the Basics of Data for You
LISTING 8.3: Testing out the User data class
The Basics of Data Includes hashCode() and equals(x)
LISTING 8.4: A non–data class version of User
LISTING 8.5: Comparing what you get “for free” between User and SimpleUser
DESTRUCTURING DATA THROUGH DECLARATIONS
Grab the Property Values from a Class Instance
Destructuring Declarations Aren't Particularly Clever
Kotlin Is Using componentN() Methods to Make Declarations Work
You Can Add componentN() Methods to Any Class
LISTING 8.6: Adding componentN() methods to SimpleUser
If You Can Use a Data Class, You Should
YOU CAN “COPY” AN OBJECT OR MAKE A COPY OF AN OBJECT
Using = Doesn't Actually Make a Copy
LISTING 8.7: Using = instead of copy()
If You Want a Real Copy, Use copy()
LISTING 8.8: Moving from = to copy()
DATA CLASSES REQUIRE SEVERAL THINGS FROM YOU
Data Classes Require Parameters and val or var
Data Classes Cannot Be Abstract, Open, Sealed, or Inner
DATA CLASSES ADD SPECIAL BEHAVIOR TO GENERATED CODE
You Can Override Compiler-Generated Versions of Many Standard Methods
Listing 8.9: A data class with an overridden toString()
Supertype Class Functions Take Precedence
Data Classes Only Generate Code for Constructor Parameters
LISTING 8.10: Person with extra properties
LISTING 8.11: Person with extra properties, converted to a data class
LISTING 8.12: Person as a data class with a declared property
LISTING 8.13: Person as a data class with all properties accepted in the constructor
Only Constructor Parameters Are Used in equals()
LISTING 8.14: Person as a data class with a single internal property
DATA CLASSES ARE BEST LEFT ALONE
LISTING 8.15: A User data class in its simplest form
LISTING 8.16: A data class with less code to generate
9 Enums and Sealed, More Specialty Classes. WHAT'S IN THIS CHAPTER?
STRINGS ARE TERRIBLE AS STATIC TYPE REPRESENTATIONS
LISTING 9.1: Creating a user of a certain type
LISTING 9.2: A user class with a type
Strings Are Terrible Type Representations
Capitalization Creates Comparison Problems
LISTING 9.3: An updated test class that checks for admins
This Problem Occurs All the Time
String Constants Can Help … Some
LISTING 9.4: Adding a constant to the User class
COMPANION OBJECTS ARE SINGLE INSTANCE
LISTING 9.5: Current User class … which won't compile
Constants Must Be Singular
Companion Objects Are Singletons
LISTING 9.6: Adding an unnamed companion object to User
Companion Objects Are Still Objects
LISTING 9.7: Filling out the companion object for User
You Can Use Companion Objects without Their Names
Using a Companion Object's Name Is Optional
LISTING 9.8: Using helper functions in the test class
LISTING 9.9: Using helper functions and adding the companion object name
Using a Companion Object's Name Is Stylistic
Companion Object Names Are Hard
LISTING 9.10: The User object with just constants in the companion object
You Can Skip the Companion Object Name Altogether
LISTING 9.11: User with an unnamed companion object
ENUMS DEFINE CONSTANTS AND PROVIDE TYPE SAFETY
Enums Classes Provide Type-Safe Values
LISTING 9.12: A new UserType enum
LISTING 9.13: Using the UserType enum in User
LISTING 9.14: Creating users with a specific UserType
Enums Classes Are Still Classes
LISTING 9.15: Overriding a method in an enum
Enums Give You the Name and Position of Constants
Each Constant in an enum Is an Object
LISTING 9.16: Overriding a function in a particular constant object
Each Constant Can Override Class-Level Behavior
LISTING 9.17: Defining a function to be overridden by constant objects
SEALED CLASSES ARE TYPE-SAFE CLASS HIERARCHIES
LISTING 9.18: A very simple Auto class
LISTING 9.19: The beginning of an enum with makes of automobiles
Enums and Class Hierarchies Work for Shared Behavior
Sealed Classes Address Fixed Options and Non-Shared Behavior
LISTING 9.20: A simple Operation class built to be subclassed
LISTING 9.21: An empty Add class that subclasses Operation
Sealed Classes Don't Have Shared Behavior
Sealed Classes Have a Fixed Number of Subclasses
LISTING 9.22: Operation as a sealed class
Listing 9.23 Operation as a sealed class with subclasses
Subclasses of a Sealed Class Don't Always Define Behavior
when Requires All Sealed Subclasses to Be Handled
LISTING 9.24: A very simple calculator with one function
when Expressions Must Be Exhaustive for Sealed Classes
LISTING 9.25: Adding additional sealed class subclasses to the when expression
else Clauses Usually Don't Work for Sealed Classes
LISTING 9.26: Adding an else to a when
else Clauses Hide Unimplemented Subclass Behavior
LISTING 9.27: Using an else to catch any extra subclasses
LISTING 9.28: Adding subclasses to Operation
LISTING 9.29: Moving errors from compile-time to runtime
10 Functions and Functions and Functions. WHAT'S IN THIS CHAPTER?
REVISITING THE SYNTAX OF A FUNCTION
Functions Follow a Basic Formula
LISTING 10.1: A super simple Kotlin class with a super simple function
Function Arguments Also Have a Pattern
LISTING 10.2: The Person class shown in Chapter 6
Default Values in Constructors Are Inherited
Default Values in Functions Are Inherited
LISTING 10.3: An open class with an open add() function
LISTING 10.4: Extending Calculator and overriding the add() function
Default Values in Functions Cannot Be Overridden
Default Values Can Affect Calling Functions
Calling Functions Using Named Arguments Is Flexible
Function Arguments Can't Be Null Unless You Say So
LISTING 10.5: Adding a function that prints a sum to Abacus
FUNCTIONS FOLLOW FLEXIBLE RULES
Functions Actually Return Unit by Default
Functions Can Be Single Expressions
Single-Expression Functions Don't Have Curly Braces
LISTING 10.6: Changing add() to be a single expression assigned to the function
Single-Expression Functions Don't Use the return Keyword
Single-Expression Functions Can Infer a Return Type
LISTING 10.7: Revisiting type inference in Calculator
Type Widening Results in the Widest Type Being Returned
Functions Can Take Variable Numbers of Arguments
LISTING 10.8: Using varargs in a new function in Calculator
A vararg Argument Can Be Treated Like an Array
FUNCTIONS IN KOTLIN HAVE SCOPE
Local Functions Are Functions Inside Functions
Member Functions Are Defined in a Class
Extension Functions Extend Existing Behavior without Inheritance
Extend an Existing Closed Class Using Dot Notation
LISTING 10.9: A closed version of the Person class
LISTING 10.10: A test program for using extension functions
this Gives You Access to the Extension Class
LISTING 10.11: Adding an extension function to Person
FUNCTION LITERALS: LAMBDAS AND ANONYMOUS FUNCTIONS
Anonymous Functions Don't Have Names
LISTING 10.12: Creating an anonymous function
LISTING 10.13: Adding parameters and a return value to an anonymous function
You Can Assign a Function to a Variable
Executable Code Makes for an “Executable” Variable
Higher-Order Functions Accept Functions as Arguments
The Result of a Function Is Not a Function
Function Notation Focuses on Input and Output
LISTING 10.14: Testing out passing a function into a higher-order function
You Can Define a Function Inline
Lambda Expressions Are Functions with Less Syntax
LISTING 10.15: A test of both anonymous and inline functions
You Can Omit Parameters Altogether
Lambda Expressions Use it for Single Parameters
it Makes Lambdas Work More Smoothly
Lambda Expressions Return the Last Execution Result
Trailing Functions as Arguments to Other Functions
LOTS OF FUNCTIONS, LOTS OF ROOM FOR PROBLEMS
11 Speaking Idiomatic Kotlin. WHAT'S IN THIS CHAPTER?
SCOPE FUNCTIONS PROVIDE CONTEXT TO CODE
USE LET TO PROVIDE IMMEDIATE ACCESS TO AN INSTANCE
LISTING 11.1: Creating and using a Calculator instance
LISTING 11.2: Using let to scope function calls
let Gives You it to Access an Instance
The Scoped Code Blocks Are Actually Lambdas
let and Other Scope functions Are Largely about Convenience
You Can Chain Scoped Function Calls
LISTING 11.3: Chaining scope functions
An Outer it “Hides” an Inner it
Chaining Scope Functions and Nesting Scope Functions Are Not the Same
Nesting Scope Functions Requires Care in Naming
Chaining Scope Functions Is Simpler and Cleaner
Prefer Chaining over Nesting
Many Chained Functions Start with a Nested Function
You Can Scope Functions to Non-Null Results
LISTING 11.4: A simple program using code and nullable values
LISTING 11.5: A non-compilable program with null problems that need to be fixed
Accepting null Values Isn't a Great Idea
Scope Functions Give You Null Options
LISTING 11.6: Using a scope function to work around potentially null values
Scope Functions Work on Other Functions … in Very Particular Ways
LISTING 11.7: Applying a scope function to a function that might return null
LISTING 11.8: Using the ? operator to apply a scope function
WITH IS A SCOPE FUNCTION FOR PROCESSING AN INSTANCE
with Uses this as Its Object Reference
A this Reference Is Always Available
with Returns the Result of the Lambda
RUN IS A CODE RUNNER AND SCOPE FUNCTION
Choosing a Scope Function Is a Matter of Style and Preference
run Doesn't Have to Operate on an Object Instance
APPLY HAS A CONTEXT OBJECT BUT NO RETURN VALUE
apply Operates upon an Instance
apply Returns the Context Object, Not the Lambda Result
?: Is Kotlin's Elvis Operator
ALSO GIVES YOU AN INSTANCE … BUT OPERATES ON THE INSTANCE FIRST
also Is Just Another Scope Function
also Executes before Assignment
SCOPE FUNCTIONS SUMMARY
LISTING 11.9: The complete set of samples for scope functions
12 Inheritance, One More Time, with Feeling. WHAT'S IN THIS CHAPTER?
ABSTRACT CLASSES REQUIRE A LATER IMPLEMENTATION
Abstract Classes Cannot Be Instantiated
LISTING 12.1: The User data class is a class for extension
LISTING 12.2: A basic Car class
LISTING 12.3: An abstract version of the Car class
LISTING 12.4: Testing out an abstract class
Abstract Classes Define a Contract with Subclasses
LISTING 12.5: Making the single function in Car abstract
LISTING 12.6: Fulfilling the contract laid out by Car
LISTING 12.7: Instantiating Honda and using the drive() function
LISTING 12.8: Adding more details to the contract that Car defines
Abstract Classes Can Define Concrete Properties and Functions
LISTING 12.9: Setting up some concrete functions and properties in Car
LISTING 12.10: Updating Honda to fulfill the contract set out by Car
LISTING 12.11: A simple program to show off Honda
Subclasses Fulfill the Contract Written by an Abstract Class
Subclasses Should Vary Behavior
LISTING 12.12: Building another subclass of Car
The Contract Allows for Uniform Treatment of Subclasses
LISTING 12.13: An Infiniti subclass of Car
LISTING 12.14: A BMW subclass of Car
LISTING 12.15: Iterating over Cars in a list
INTERFACES DEFINE BEHAVIOR BUT HAVE NO BODY
LISTING 12.16: Building an interface for vehicles
LISTING 12.17: Implementing the Vehicle interface
Interfaces and Abstract Classes Are Similar
LISTING 12.18: An abstract class implementing an interface
Interfaces Cannot Maintain State
A Class's State Is the Values of Its Properties
An Interface Can Have Fixed Values
LISTING 12.19: Adding a property with a getter to Vehicle
Interfaces Can Define Function Bodies
Interfaces Allow Multiple Forms of Implementation
LISTING 12.20: Car as an Interface (with errors)
LISTING 12.21: A new interface for vehicle manufacturers
LISTING 12.22: Extending the Manufacturer implementation
A Class Can Implement Multiple Interfaces
LISTING 12.23: Honda can extend a class and implement interfaces
Interface Property Names Can Get Confusing
Interfaces Can Decorate a Class
LISTING 12.24: Defining a new function on Manufacturer implementations
LISTING 12.25: Adding behavior available to all implementations of the interface
DELEGATION OFFERS ANOTHER OPTION FOR EXTENDING BEHAVIOR
Abstract Classes Move from Generic to Specific
LISTING 12.26 A MODIFIED VEHICLE INTERFACE WITH A MORE GENERIC NAME FOR MOVING
LISTING 12.27: Updating Car to pass go() calls to drive()
More Specificity Means More Inheritance
LISTING 12.28: The Motorcycle class is a close analog to the Car class
LISTING 12.29: One concrete subclass of Motorcycle: a Harley
LISTING 12.30: Another subclass of Motorcycle, this time a Honda
LISTING 12.31: A Plane implementation of Vehicle
LISTING 12.32: A Plane subclass: the B52!
LISTING 12.33: One More Plane: a hang glider
Delegating to a Property
LISTING 12.34: The Spy class, using several vehicles
LISTING 12.35: Delegating Vehicle calls to a property
LISTING 12.36: A test program for the delegating Spy
Delegation Occurs at Instantiation
LISTING 12.37: Delegating to three different interfaces
INHERITANCE REQUIRES FORETHOUGHT AND AFTERTHOUGHT
13 Kotlin: The Next Step. WHAT'S IN THIS CHAPTER?
PROGRAMMING KOTLIN FOR ANDROID
Kotlin for Android Is Still Just Kotlin
LISTING 13.1: Loading and displaying text on an initial view
Move from Concept to Example
KOTLIN AND JAVA ARE GREAT COMPANIONS
Your IDE Is a Key Component
LISTING 13.2: Interacting with Java from Kotlin
LISTING 13.3: Using the Kotlin class that in turn uses Java
Kotlin Is Compiled to Bytecode for the Java Virtual Machine
Gradle Gives You Project Build Capabilities
WHEN KOTLIN QUESTIONS STILL EXIST
Use the Internet to Supplement Your Own Needs and Learning Style
NOW WHAT?
INDEX
WILEY END USER LICENSE AGREEMENT
Отрывок из книги
Brett McLaughlin
Then I wrote a book. Now I understand.
.....
One of the cool things about a class method is that you can write code and define what that method does. We haven't done that yet, but it's coming soon. In the meantime, though, what we have here is slightly different: a method that we didn't write code for, and that doesn't do what we want.
In this case, you can do something called overriding a method. This just means replacing the code of the method with your own code. That's exactly what we want to do here.
.....