PHP This! A Beginners Guide to Learning Object Oriented PHP
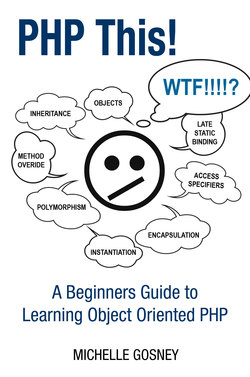
Реклама. ООО «ЛитРес», ИНН: 7719571260.
Оглавление
Michelle Gosney. PHP This! A Beginners Guide to Learning Object Oriented PHP
Introduction
Why Read This Book
Why Learn Object Oriented PHP
Chapter 1: PHP Objects & Classes. Initial Confusion
PHP Objects & Classes
Instantiation
$this Variable
Access Modifiers
Inheritance
Method Overriding
Invoking Parent Methods
Horizontal Inheritance – Using Traits
Encapsulation
Polymorphism
Method Overloading
Method Overriding
Late Binding / Dynamic Binding
Chapter 2: Magic Methods
__construct()
Invoking Parent Constructor and Destructor
__destruct()
__get(), __set(), __isset()
__call()
__sleep()
__wakeup()
__clone()
Chapter 3: Abstract Classes & Methods
abstract Keyword
Abstract Methods
final Keyword
Chapter 4: PHP Interfaces
interface & implements Keywords
Chapter 5: The static Modifier
Static Properties
Singleton Pattern
Late Static Binding
Chapter 6: PHP Error Control & Exception Handling
The Built-in Exception Class
Throwing an Exception
Setting the Desired Error Sensitivity Level
Error Reporting Sensitivity Levels
Displaying Startup Errors
Logging Errors
Identifying the Log File
Setting the Maximum Log Line Length
Ignoring Repeated Errors
Ignoring Errors Originating from the Same Location
Storing Most Recent Error in a Variable
Opening the Logging Connection
Logging Options
Closing the Logging Connection
Chapter 7: Model – View – Controller. Understanding the Model-View-Controller Design Pattern
Model
View
Controller
The MVC URL Structure & URL Mapping
The index.php File
MVC Folder Structure
View
The Display Class
Controller
Model
Develop the Functionality to Add New Menu Items
Controller for the Add Menu Item Feature
Model for the Add Menu Item Feature
View for the Add MenuItem Feature
View Templates for the Add MenuItem Feature
Display Manager for the Add MenuItem Feature
View Templates for the Assign MenuItem-to-Menu Feature
Controller for the Assign MenuItem-to-Menu Feature
Model for the Assign MenuItem-to-Menu Feature
Display Manager for the Assign MenuItem-to-Menu View
Controller for the Edit/Delete MenuItem Feature
Model for the Edit MenuItem Feature
General Markup for the Edit MenuItem Page
View Templates for the Edit MenuItem Feature
Display Manager for the Edit View
Chapter 8: The Reflection API
Chapter 9: The PEAR Library
Install PEAR in a Windows Zend Framework Environment
Install PEAR in a Windows XAMPP Environment
Install PEAR in a Windows WAMP Environment
Install PEAR on Mac OS
Update System PATH
Update PHP Include Path
Install PEAR on Linux
Using PEAR - EXAMPLE. Text -> CAPTCHA_Numeral
Chapter 10: Unit Testing with PHPUnit
Installing PHPUnit
Install PHPUnit on Windows on Zend Framework Environment
Install PHPUnit on Windows on XAMPP Environment
Install PHPUnit on Mac OS X
Install PHPUnit on Linux
Test Fixtures
Test Phases
Writing Test Cases
Assertion Methods
Testing Data from HTML Form Submissions
Test Suites
Mocks and Stubs
Create a Mock Class
Running PHPUnit from Eclipse Without Plug-ins
Chapter 11: Source Code Management with Subversion
Setting Up a Subversion Server and Client on Windows
Adding Your Source Code the First Time
Creating a Copy to Work On
Updating and Committing Your Working Copy
Setting up TortoiseSVN
Exporting a Release
Tagging & Branching a File
Setting Up a Subversion Server and Client on Mac OS X. Download Subversion
Unpack and Install Subversion
Add Subversion to Your Path
Setting up the Subversion Repository
Creating a Subversion Project
Checking Out Files from Subversion
Updating and Committing Changes
Adding and Removing Files and Directories. Adding a File
Removing a File
Adding a Directory
Removing a Directory
Tagging & Exporting a Release
Branching & Merging a Project. Creating a Branching
Merging
Graphical User Interfaces
Setting Up a Subversion Server on a Linux Server
Step 1:
Step 2:
Step 3:
Step 4:
Step 5:
Step 6:
Step 7:
Updating and Committing Changes
Adding and Removing Files and Directories. Adding a File
Removing a File
Adding a Directory
Removing a Directory
Tagging & Exporting
Branching & Merging a Project. Creating a Branching
Merging
Summary
Appendix A: Bibliography. Web Resources
Books
Appendix B: Setting Up Your Development Stack
Install Zend Server CE (Community Edition)
MySQL Workbench 5.2 CE
Setting Up Your Integrated Development Environment
Отрывок из книги
A few years ago when I first sat down to evaluate the new features of PHP 5.3 I knew I was facing a challenging learning curve. It had been about 9 years since I had used PHP and at that time it was an easy to use, loosely typed, scripting language. My first thought while exploring the new features of PHP 5.3 was that “wow, little PHP is all grown up.”
In an effort to get caught up in a hurry, I looked at several books and on-line forums and quickly grew frustrated with the same boring and monotonous way that these advanced concepts were explained and the poor examples that were used. Having an extensive background in object-oriented programming I continuously thought to myself “what if someone is trying to learn these concepts without much of an object-oriented background?”
.....
So to summarize the three PHP access Modifiers in a more formal way:
private restricts the access to the class itself. Only methods that are part of the same class can access private members. Let’s look at an example below:
.....