Data Structure and Algorithms Using C++
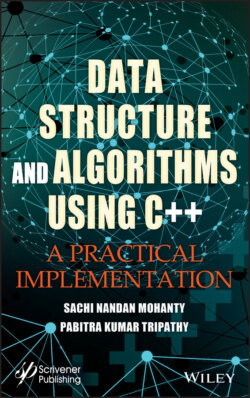
Реклама. ООО «ЛитРес», ИНН: 7719571260.
Оглавление
Sachi Nandan Mohanty. Data Structure and Algorithms Using C++
Table of Contents
Guide
Pages
Data Structure and Algorithms Using C++ A Practical Implementation
Preface
1. Introduction to Data Structure. 1.1 Definition and Use of Data Structure
1.2 Types of Data Structure
1.3 Algorithm
1.4 Complexity of an Algorithm
1.5 Efficiency of an Algorithm
1.6 Asymptotic Notations
1.7 How to Determine Complexities
1.8 Questions
2. Review of Concepts of ‘C++’ 2.1 Array
2.1.1 One-Dimensional Array
2.1.2 Multi-Dimensional Array
2.1.3 String Handling
2.2 Function
2.2.1 User Defined Functions
2.2.2 Construction of a Function
2.2.3 Actual Argument and Formal Argument
2.2.4 Call by Value and Call by Reference
2.2.5 Default Values for Parameters
2.2.6 Storage Class Specifiers
2.3 Pointer
2.3.1 Declaration of a Pointer
2.3.2 Initialization of a Pointer
2.3.3 Arithmetic With Pointer
2.3.4 Passing of a Pointer to Function
2.3.5 Returning of a Pointer by Function
2.3.6 C++ Null Pointer
2.4 Structure
2.4.1 The typedef Keyword
2.5 Questions
3. Sparse Matrix. 3.1 What is Sparse Matrix
3.2 Sparse Matrix Representations
3.3 Algorithm to Represent the Sparse Matrix
3.4 Programs Related to Sparse Matrix
3.5 Why to Use Sparse Matrix Instead of Simple Matrix?
3.6 Drawbacks of Sparse Matrix
3.7 Sparse Matrix and Machine Learning
3.8 Questions
4. Concepts of Class. 4.1 Introduction to CLASS
4.2 Access Specifiers in C++
4.3 Declaration of Class
4.4 Some Manipulator Used In C++
4.5 Defining the Member Functions Outside of the Class
4.6 Array of Objects
4.7 Pointer to Object
4.8 Inline Member Function
4.9 Friend Function
4.9.1 Simple Friend Function
4.9.2 Friend With Inline Substitution
4.9.3 Granting Friendship to Another Class (Friend Class)
4.9.4 More Than One Class Having the Same Friend Function
4.10 Static Data Member and Member Functions
4.11 Constructor and Destructor. 4.11.1 Constructor
4.11.1.1 Empty Constructor
4.11.1.2 Default Constructor
4.11.1.3 Parameterized Constructors
4.11.1.4 Copy Constructor
4.11.2 Destructor
4.12 Dynamic Memory Allocation
4.13 This Pointer
4.14 Class Within Class
4.15 Questions
5. Stack. 5.1 STACK
5.2 Operations Performed With STACK
5.3 ALGORITHMS
5.4 Applications of STACK
5.5 Programming Implementations of STACK
5.6 Questions
6. Queue. 6.1 Queue
6.2 Types of Queue
6.3 Linear Queue OVERFLOW
6.4 Circular Queue
6.5 Double Ended Queue
6.6 Priority Queue
6.7 Programs
6.8 Questions
7. Linked List
7.1 Why Use Linked List?
7.2 Types of Link List
7.3 Single Link List
7.4 Programs Related to Single Linked List. 7.4.1 /* Creation of a Linked List */
7.4.2 /* Insert a Node Into a Simple Linked List at the Beginning */
7.4.3 /* Insert a Node Into a Simple Linked List at the End of the List */
7.4.4 /* Insert a Node Into a Simple Linked List When the Node Is Known */
7.4.5 /* Insert a Node Into a Simple Linked List Information Is Known and Put After Some Specified Node */
7.4.6 /* Deleting the First Node From a Simple Linked List */
7.4.7 /* Deleting the Last Node From a Simple Linked List */
7.4.8 /* Deleting a Node From a Simple Linked List When Node Number Is Known */
7.4.9 Deleting a Node From a Simple Linked List When Information of a Node Is Given
7.4.10 /* SEARCH A NODE INTO A SIMPLE LINKED LIST WITH INFORMATION IS KNOWN */
7.4.11 /* Sorting a Linked List in Ascending Order */
7.4.12 /* Reversing a Linked List */
7.4.13 Program for Student Data Using Linked List
7.5 Double Link List
7.6 Programs on Double Linked List. 7.6.1 /* Creation of Double Linked List */
7.6.2 /* Inserting First Node in the Doubly Linked List */
7.6.3 /*Inserting a Node in the Doubly Linked List When Node Number Is Known*/
7.6.4 /*Inserting a Node in the Doubly Linked List When Information Is Known*/
7.6.5 /* Delete First Node From a Double Linked List */
7.6.6 /*Delete the Last Node From the Double Linked List*/
7.7 Header Linked List
7.7.1 /* Inserting a Node Into a Header Linked List */
7.8 Circular Linked List
7.9 Application of Linked List
7.9.1 Addition of Two Polynomial
7.9.2 /* Polynomial With Help of Linked List */
7.9.3 Program for Linked Queue
7.9.4 Program for Linked Stack
7.10 Garbage Collection and Compaction
7.11 Questions
8. TREE
8.1 Tree Terminologies
8.2 Binary Tree
8.3 Representation of Binary Tree
8.3.1 Array Representation of a Tree
8.3.2 Linked List Representation of a Tree
8.4 Operations Performed With the Binary Tree
8.4.1 /*Creation of a Tree*/
8.5 Traversing With Tree
8.5.1 /* Binary Tree Traversal */
8.6 Conversion of a Tree From Inorder and Preorder
8.7 Types of Binary Tree
8.8 Expression Tree
8.9 Binary Search Tree
8.10 Height Balanced Tree (AVL Tree)
8.11 Threaded Binary Tree
8.12 Heap Tree
8.13 Huffman Tree
8.14 Decision Tree
8.15 B-Tree
8.16 B + Tree
8.17 General Tree
8.18 Red–Black Tree
8.19 Questions
9. Graph
9.1 Graph Terminologies DIRECTED GRAPH
9.2 Representation of Graph
9.3 Traversal of Graph
9.3.1 Breadth First Search (BFS)
9.3.2 Depth First Search
9.4 Spanning Tree
9.4.1 Kruskal Algorithm
9.4.2 Prim’s Algorithm
9.5 Single Source Shortest Path
9.5.1 Bellman–Ford Algorithm
9.5.2 Dijkstra’s Algorithm
9.6 All Pair Shortest Path
9.7 Topological Sorting
9.8 Questions
10. Searching and Sorting
10.1 Linear Search
10.2 Binary Search
10.3 Bubble Sort
10.4 Selection Sort
10.5 Insertion Sort
10.6 Merge Sort
10.7 Quick Sort
10.8 Radix Sort
10.9 Heap Sort
10.10 Questions
11. Hashing
11.1 Hash Functions
11.2 Collisions
11.3 Collision Resolution Methods Linear Probing
11.4 Clustering
11.5 Questions
Index
WILEY END USER LICENSE AGREEMENT
Отрывок из книги
Scrivener Publishing 100 Cummings Center, Suite 541J Beverly, MA 01915-6106
.....
This notation bounds the function to within constant factors. We say f(n) = θg(n) if there exists +ve constants n0, C1 and C2 such that to the right of n0 the value of f(n) always lies between c1g(n) and c2(g(n)) inclusive.
Little Oh Notation (o)
.....